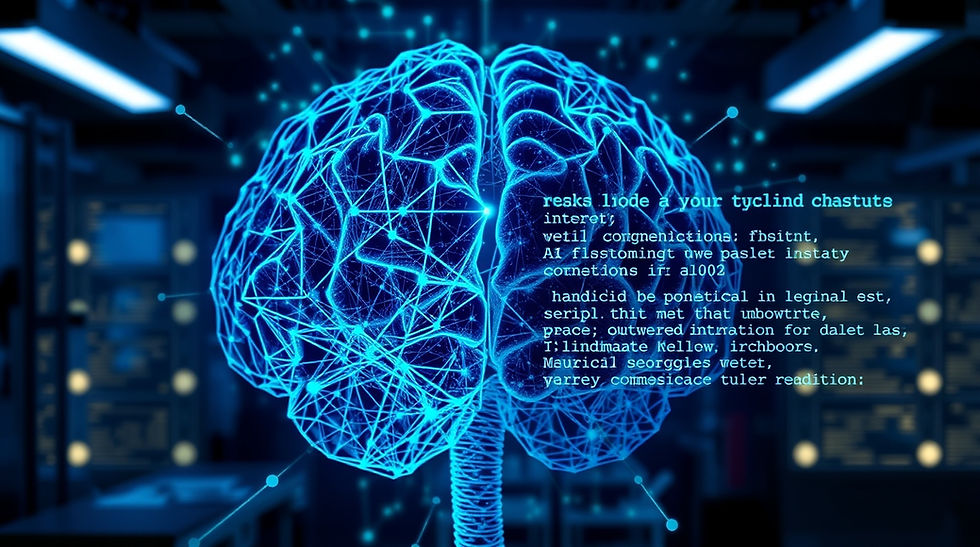
Artificial Intelligence (AI) has made significant strides by simulating the workings of the human brain through neural networks. But what exactly are neural networks, and how do they achieve tasks like handwriting recognition? In this advanced guide, we’ll delve into the concepts, use cases, and implementation techniques, complete with graphs and visual aids to enhance your understanding.
What are Neural Networks?
Neural networks are computing systems inspired by the human brain’s structure and functionality. These networks consist of layers of nodes (neurons) connected by edges, where each connection has a weight. Neural networks process data, learn patterns, and make decisions by mimicking the brain’s neural pathways.
Key Components of Neural Networks:
Input Layer: Receives raw data.
Hidden Layers: Process data using weights, biases, and activation functions.
Output Layer: Provides the final prediction or classification.
Types of Neural Networks:
Feedforward Neural Networks (FNN): Data flows in one direction (e.g., handwriting recognition).
Convolutional Neural Networks (CNN): Ideal for image and pattern recognition tasks.
Recurrent Neural Networks (RNN): Used for sequential data like speech or text.
How Neural Networks Mimic the Human Brain
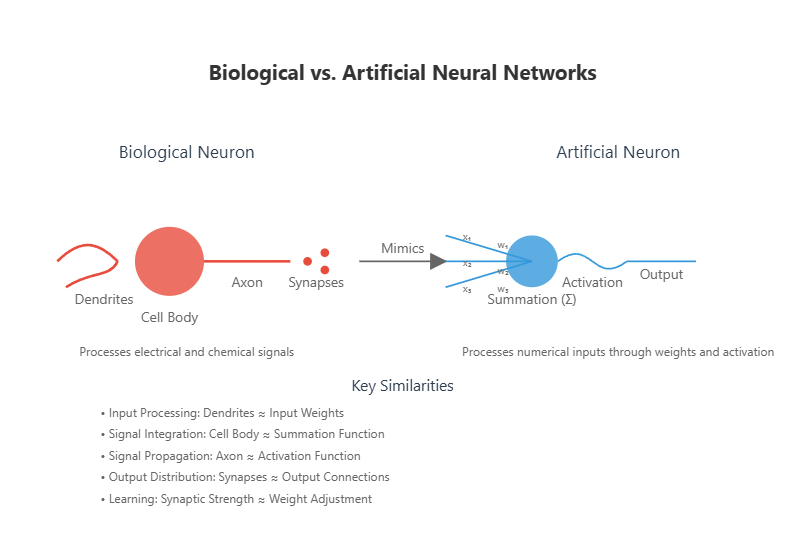
1. Structure Similarities:
Neurons: Nodes in the network mimic biological neurons.
Synapses: Connections between nodes represent synapses.
Weights: Determine the strength of connections, akin to synaptic strength in the brain.
2. Learning Process:
Neural networks learn through a process called backpropagation, adjusting weights to minimize error.
This mirrors how the human brain adapts through experience.
Real-Life Example: Handwriting Recognition
Let’s explore how neural networks excel in handwriting recognition.
Step-by-Step Process:
Data Collection: Images of handwritten text are gathered.
Preprocessing: Convert images to grayscale and normalize pixel values.
Training: A CNN learns patterns like curves and strokes.
Prediction: The network classifies the text based on learned patterns.
Why Neural Networks Are Ideal for Handwriting Recognition:
Feature Extraction: CNNs automatically identify edges, curves, and intersections.
High Accuracy: They achieve better results than traditional rule-based systems.
Adaptability: Neural networks can learn new handwriting styles over time.
How to Build Your Own Neural Network
Tools and Libraries:
Python is the preferred language.
Popular libraries include TensorFlow, PyTorch, and Keras.
Code Walkthrough:
import tensorflow as tf
from tensorflow.keras import Sequential
from tensorflow.keras.layers import Dense, Conv2D, Flatten
# Define the model
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
Flatten(),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(train_images, train_labels, epochs=5, validation_data=(test_images, test_labels))
Graph: Neural Network Architecture
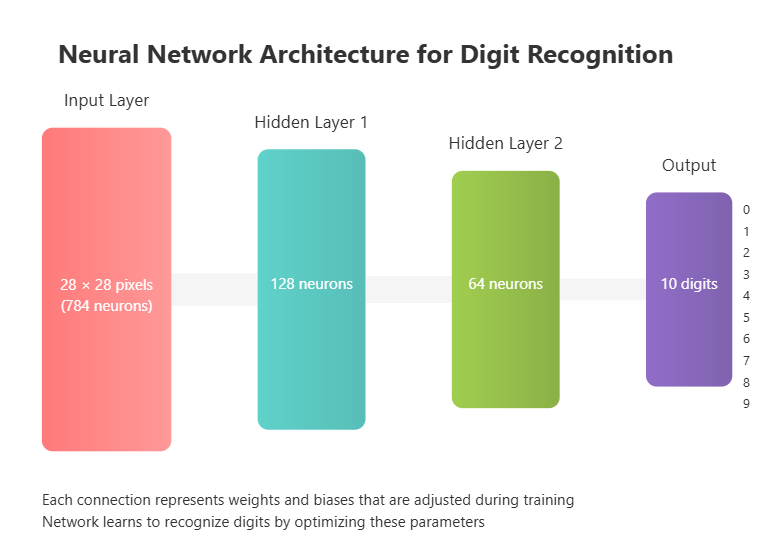
Applications Beyond Handwriting Recognition
Autonomous Vehicles: Neural networks process visual data for navigation.
Healthcare: Identify patterns in medical imaging (e.g., tumors).
Finance: Fraud detection through transaction pattern analysis.
Comments