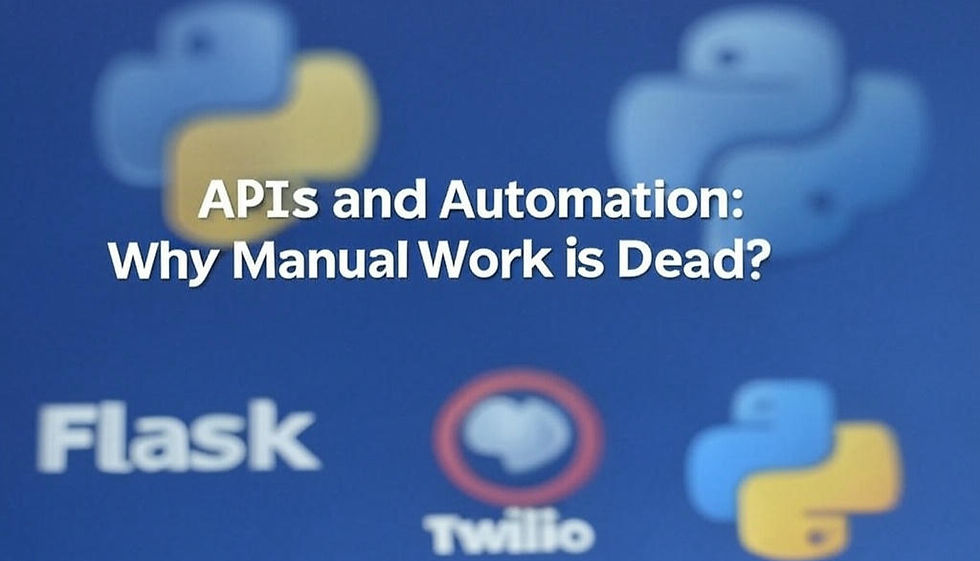
Welcome Back to Day 2!
Hey, beginner! Yesterday, on Day 1, we dipped our toes into APIs by grabbing the weather for New York using Python and the OpenWeatherMap API. Pretty neat, right? Today, we’re stepping it up: we’ll automate sending that weather update to your friend via text message. No more manual copying and pasting—just pure, hands-off tech magic. Let’s see how APIs and automation can make your mornings even smoother!
Quick Recap: What We Did on Day 1
We wrote a simple script that fetched the weather—like a digital barista handing us the forecast. It printed something like:Good morning! The weather in New York is 42°F with cloudy skies.Today, we’ll take that data and send it to your friend’s phone automatically, using an API for texting.
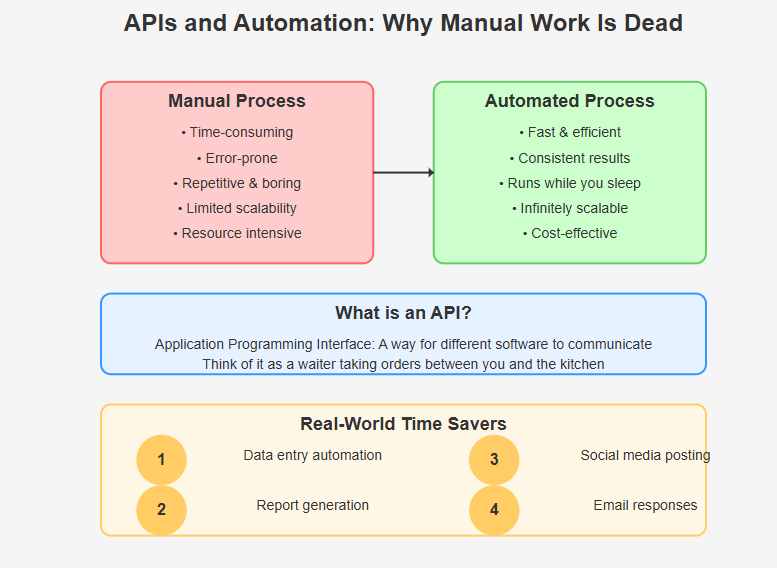
Real-Life Example: Texting Your Friend the Weather
Imagine your friend always forgets to check the weather and ends up soaked without an umbrella. You’ve been their weather hero, but texting manually every day is a chore. Let’s automate it! Here’s the plan:
Use the OpenWeatherMap API to get the weather (like yesterday).
Use the Twilio API to send a text message with that weather info.
Run it automatically every morning.
By the end, your friend will get a text like:“Good morning! Today in New York: 42°F, cloudy skies. Bring an umbrella!”
Step 1: Setting Up Twilio
Twilio is a service that lets you send texts via an API. Here’s how to get started:
Sign up for a free Twilio account at twilio.com.
Get a Twilio phone number (they give you one in the trial).
Grab your Account SID, Auth Token, and Twilio phone number from your dashboard—we’ll need these for the code.
Your Day 2 Code: Weather + Texting
We’ll build on yesterday’s script and add Twilio to send the message. You’ll need:
Python and the requests library (from Day 1).
The twilio library—install it with pip install twilio in your terminal.
Here’s the code:
python
CollapseWrapCopy
import requests # For the weather API
from twilio.rest import Client # For sending texts # Weather API setup (OpenWeatherMap) api_key = "YOUR_OPENWEATHERMAP_API_KEY" # Replace with your key
city = "New York" url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=imperial"
response = requests.get(url) data = response.json() temp = data["main"]["temp"] description = data["weather"][0]["description"] weather_message = f"Good morning! Today in {city}: {temp}°F, {description}." # Twilio setup account_sid = "YOUR_TWILIO_ACCOUNT_SID" # Replace with your SID auth_token = "YOUR_TWILIO_AUTH_TOKEN" # Replace with your token twilio_number = "YOUR_TWILIO_PHONE_NUMBER" # Replace with your Twilio number friend_number = "YOUR_FRIENDS_PHONE_NUMBER" # Replace with their number (e.g., "+11234567890") # Connect to Twilio and send the message client = Client(account_sid, auth_token) message = client.messages.create( body=weather_message, from_=twilio_number, to=friend_number ) print("Text sent! Here’s what your friend got:") print(weather_message)
How It Works
Weather Part: Same as Day 1—fetches the temperature and description from OpenWeatherMap.
Twilio Part:
account_sid and auth_token are your Twilio credentials.
twilio_number is the number Twilio gave you.
friend_number is your friend’s number (include the country code, like +1 for the US).
client.messages.create() sends the text with your weather message.
Output: It prints what was sent, so you can confirm it worked.
Try It Out!
Install twilio with pip install twilio.
Replace the placeholders (YOUR_OPENWEATHERMAP_API_KEY, YOUR_TWILIO_ACCOUNT_SID, etc.) with your real values.
Save it as weather_text.py and run it with python weather_text.py.
If everything’s set up, your friend gets a text, and you’ll see the message printed in your terminal. (Note: Twilio’s free tier requires your friend’s number to be verified in their dashboard—add it there first!)
Step 3: Automating It
To make this run every morning:
Windows: Use Task Scheduler to run python weather_text.py at 7 AM.
Mac/Linux: Use cron. Open a terminal and type crontab -e, then add:
0 7 * /usr/bin/python3 /path/to/weather_text.py
(Adjust the path to your script.)
Now, your friend gets their weather update without you lifting a finger!
Why This Matters
APIs: You’ve now used two—OpenWeatherMap for data and Twilio for action.
Automation: Scheduling turns a one-time script into a daily helper.
Real Life: This saves you time and makes you a tech-savvy friend!
Day 2 Takeaways
APIs can fetch data and perform actions like texting.
With a few lines of code, you’ve automated a real task.
You’re starting to see how tech can work for you.
What’s Next?
Tomorrow, we could:
Add more APIs (like news headlines with the weather).
Explore no-code tools like Zapier for automation.
Try a new project—like automating coffee shop inventory alerts.
What do you think—any ideas for Day 3?
Notes for You
Code: It’s beginner-friendly and builds on Day 1. Twilio’s free tier has limits (e.g., verified numbers only), but it’s perfect for learning.
Real-Life Focus: The texting example is practical and relatable, showing automation’s value.
Tone: Casual and encouraging—let me know if you want adjustments!
Next Steps: I suggested Day 3 ideas. Pick one, or let me know what you’d like!
Comments