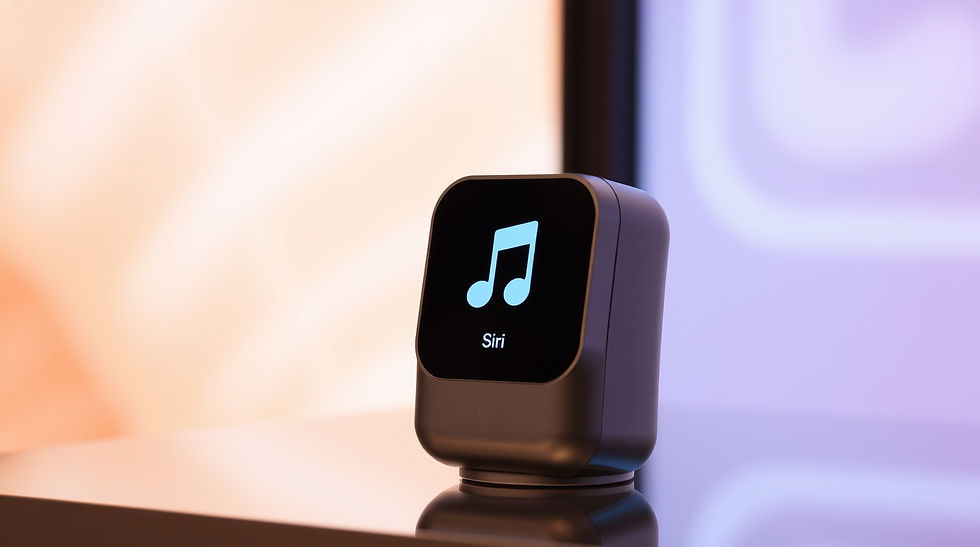
What is Artificial Intelligence?
Artificial Intelligence (AI) is a branch of computer science that aims to create intelligent machines that can perform tasks that typically require human intelligence. Think of it as teaching computers to think, learn, and make decisions, similar to how humans do.
Core Components of AI
Input Processing: Ability to receive and understand various forms of data (text, voice, images)
Learning: Capacity to learn from patterns in data
Decision Making: Capability to make informed decisions based on learned patterns
Output Generation: Ability to produce human-understandable responses
Real-Life Example: How Siri/Alexa Works
Let's break down how AI voice assistants work:
# Simplified example of how voice assistants process commands
# Simplified example of how voice assistants process commands
class VoiceAssistant:
def init(self): self.wake_word = "hey siri" # or "alexa"
self.commands_db = { "what's the weather": self.get_weather,
"set an alarm": self.set_alarm,
def listen(self, audio_input): # Convert audio to text using Speech Recognition text = speech_to_text(audio_input)
if self.wake_word in text.lower():
return self.process_command(text)
return None
def process_command(self, text): # Natural Language Processing to understand intent command = extract_intent(text)
if command in self.commands_db:
return self.commands_db[command]()
else: return "I'm sorry, I didn't understand that command."
def get_weather(self):
return "Fetching weather data..."
def set_alarm(self):
return "Setting alarm..."
def play_music(self):
return "Playing your favorite music..." # Example usage assistant = VoiceAssistant() response = assistant.listen("Hey Siri, what's the weather like today?")
print(response) # Output: "Fetching weather data..."
How AI Voice Assistants Process Information
Voice Input
Microphone captures your voice
Audio is converted to digital signal
Wake word detection ("Hey Siri" or "Alexa")
Processing
Speech-to-text conversion
Natural Language Processing (NLP) to understand meaning
Context analysis
Intent recognition
Response Generation
Query relevant databases/APIs
Generate appropriate response
Convert text to speech
Output through speakers
Real-World Applications of Voice Assistants
Home Automation
# Example of home automation command
def control_smart_home(command):
if "lights" in command:
return toggle_lights()
elif "temperature" in command:
return adjust_thermostat()
Information Retrieval
# Example of answering questions
def answer_question(query):
# Search knowledge base
relevant_info = search_database(query)
return format_response(relevant_info)
Key Technologies Behind Voice Assistants
Speech Recognition
Natural Language Processing
Machine Learning
Text-to-Speech Synthesis
Limitations and Challenges
Understanding different accents and languages
Background noise interference
Context understanding
Privacy concerns
Practice Exercise
Try creating a simple command processor:
pythonCopydef basic_command_processor(command):
commands = {
"hello": "Hi there!",
"time": "Current time is...",
"help": "Available commands: hello, time, help"
}
return commands.get(command.lower(), "Command not recognized")
# Test it
print(basic_command_processor("hello")) # Output: "Hi there!"
Next Steps
Tomorrow we'll dive deeper into machine learning, the backbone of modern AI systems. We'll explore how AI systems learn from data and make predictions.
Comments