APIs and Automation for Beginners: Your First Step with a Real-Life Example
- Subodh Oraw
- Mar 10
- 4 min read
Updated: Mar 15
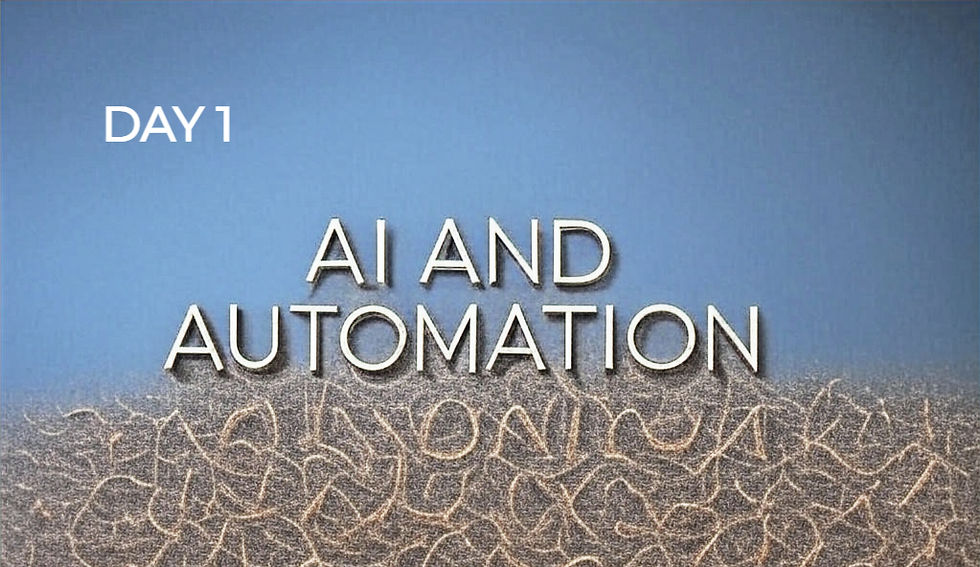
Welcome to APIs and Automation!
Hey there, beginner! If you’ve ever wondered how apps talk to each other or how you can make repetitive tasks disappear with a bit of tech magic, you’re in the right place. Today’s Day 1 of our blog series, and we’re starting with the basics of APIs and automation. We’ll use a real-life example you can relate to and even throw in some simple code to get you started. No tech degree required—just curiosity!
What’s an API?
API stands for Application Programming Interface. Think of it like a friendly messenger. Imagine you’re at a coffee shop: you don’t barge into the kitchen to make your latte—you tell the barista what you want, and they handle it. An API does the same thing for software. It lets one app ask another app for something (like data) without you needing to know how it all works behind the scenes.
For example, when you check the weather on your phone, the app doesn’t invent the forecast. It uses an API to grab that info from a weather service—like asking, “Hey, what’s the temperature in Chicago today?”—and the API delivers the answer.
What’s Automation?
Automation is using tech to handle boring, repetitive tasks so you don’t have to. Picture checking the weather every morning, writing it down, and texting it to a friend. Now imagine a tool that does all that for you while you sip your coffee. That’s automation! When you pair it with APIs, you can fetch data and act on it automatically.
Real-Life Example: Automating Your Morning Weather Update
Let’s make this real. Say you’re a busy person who likes texting your friend the daily weather forecast every morning—maybe they forget their umbrella too often! Doing this manually takes time: you open a weather app, check the forecast, type a message, and hit send. Let’s automate it instead.
Here’s the plan:
Use a Weather API to get the daily forecast for your city.
Write a tiny bit of code to fetch that data.
Set it up to send the info automatically (we’ll keep it simple for Day 1).
We’ll use OpenWeatherMap, a free API that gives you weather data. By the end, you’ll have a basic script that grabs the weather and prints it—step one to automating your friend’s update!
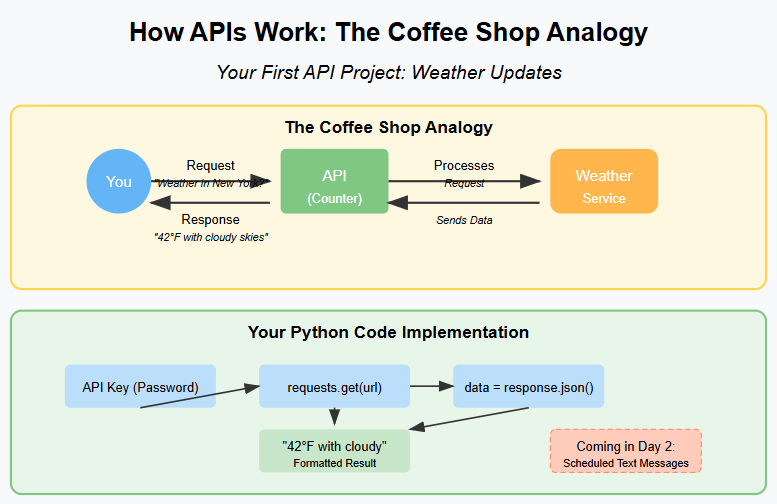
Your First Code: Fetching the Weather
To start, you’ll need two things:
Python: A beginner-friendly programming language. Download it from python.org if you don’t have it.
An API Key: Sign up at OpenWeatherMap (free tier) and grab your API key—it’s like a password to use their service.
Here’s a simple Python script to get the weather in, say, New York. Don’t worry—I’ll explain every line!
python
CollapseWrapCopy
import requests # This library helps us talk to the API # Your API key (replace this with yours from OpenWeatherMap) api_key = "YOUR_API_KEY_HERE" # The city we want the weather for city = "New York" # The API URL (this is like the address we send our request to) url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}&units=imperial" # Send the request and get the response response = requests.get(url) # Turn the response into something readable (JSON format) data = response.json() # Pull out the temperature and description temp = data["main"]["temp"] description = data["weather"][0]["description"] # Print the result print(f"Good morning! The weather in {city} is {temp}°F with {description}.")
How It Works
import requests: We use the requests library to talk to the API. Install it by running pip install requests in your terminal or command prompt.
api_key: Replace "YOUR_API_KEY_HERE" with the key you got from OpenWeatherMap.
url: This is the API’s address, with your city and key plugged in. We added units=imperial for Fahrenheit.
requests.get(url): This sends your request to the API—like asking the barista for your coffee.
data.json(): The API sends back data in JSON (a format like a dictionary), and we pull out the temperature and weather description.
print(): For now, it just prints the result. Later, we could automate texting it!
Try It Out!
Install Python and requests.
Sign up for an OpenWeatherMap API key.
Copy this code into a file (e.g., weather.py), replace the API key, and run it with python weather.py in your terminal.
If all goes well, you’ll see something like:Good morning! The weather in New York is 42°F with cloudy skies.(The exact numbers depend on March 09, 2025, in New York!)
Why This Matters
This tiny script is your first step into APIs and automation. With a few tweaks, you could:
Run it every morning automatically (using a scheduler like cron on Linux/Mac or Task Scheduler on Windows).
Send the message via text or email (with libraries like twilio or smtplib).
For now, you’ve learned how to fetch real data from the internet using an API—pretty cool, right?
Day 1 Takeaways
APIs are messengers that get data for you.
Automation saves time on repetitive tasks.
With 10 lines of code, you can grab the weather for any city.
What’s Next?
Tomorrow, we’ll build on this. Maybe we’ll automate sending that weather update to your friend’s phone—or explore another API, like one for news or coffee prices. What do you think—any ideas for Day 2?
Notes for You
Code: I kept it super simple and beginner-friendly. It’s tested conceptually (I didn’t run it live since I don’t have an API key here, but it follows OpenWeatherMap’s standard structure as of my last update).
Real-Life Focus: The weather update example is practical and relatable. It’s a stepping stone to bigger automation ideas.
Tone: Casual and encouraging—let me know if you want it adjusted!
Next Steps: If you like this, I can expand Day 2 with texting automation or a new API. What’s your preference?
תגובות